153f77827dd75fc74aff90838d0f6b3955c1b27d,sk_dsp_comm/digitalcom.py,,QAM_SEP,#Any#Any#Any#Any#Any#Any#,423
Before Change
rx_data = np.rint((M-1)*(rx_data + (1+1j))/2.)
// Fix-up edge points real part
s1r = mlab.find(rx_data.real > M - 1)
s2r = mlab.find(rx_data.real < 0)
rx_data.real[s1r] = (M - 1)*np.ones(len(s1r))
rx_data.real[s2r] = np.zeros(len(s2r))
// Fix-up edge points imag part
s1i = mlab.find(rx_data.imag > M - 1)
s2i = mlab.find(rx_data.imag < 0)
rx_data.imag[s1i] = (M - 1)*np.ones(len(s1i))
rx_data.imag[s2i] = np.zeros(len(s2i))
rx_data = 2*rx_data - (M - 1)*(1 + 1j)
//Correlate the first Ncorr symbols at four possible phase rotations
R0,lags = xcorr(rx_data,tx_data,Ncorr)
R1,lags = xcorr(rx_data*(1j)**1,tx_data,Ncorr)
R2,lags = xcorr(rx_data*(1j)**2,tx_data,Ncorr)
R3,lags = xcorr(rx_data*(1j)**3,tx_data,Ncorr)
//Place the zero lag value in the center of the array
R0max = np.max(R0.real)
R1max = np.max(R1.real)
R2max = np.max(R2.real)
R3max = np.max(R3.real)
R = np.array([R0max,R1max,R2max,R3max])
Rmax = np.max(R)
kphase_max = np.where(R == Rmax)[0]
kmax = kphase_max[0]
//Find correlation lag value is zero at the center of the array
if kmax == 0:
lagmax = lags[np.where(R0.real == Rmax)[0]]
elif kmax == 1:
lagmax = lags[np.where(R1.real == Rmax)[0]]
elif kmax == 2:
lagmax = lags[np.where(R2.real == Rmax)[0]]
elif kmax == 3:
lagmax = lags[np.where(R3.real == Rmax)[0]]
taumax = lagmax[0]
if SEP_disp:
print("Phase ambiquity = (1j)**%d, taumax = %d" % (kmax, taumax))
//Count symbol errors over the entire input ndarrays
//Begin by making tx and rx length equal and apply
//phase rotation to rx_data
if taumax < 0:
tx_data = tx_data[-taumax:]
tx_data = tx_data[:min(len(tx_data),len(rx_data))]
rx_data = (1j)**kmax*rx_data[:len(tx_data)]
else:
rx_data = (1j)**kmax*rx_data[taumax:]
rx_data = rx_data[:min(len(tx_data),len(rx_data))]
tx_data = tx_data[:len(rx_data)]
//Convert QAM symbol difference to symbol errors
errors = np.int16(abs(rx_data-tx_data))
// Detect symbols errors
// Could decode bit errors from symbol index difference
idx = mlab.find(errors != 0)
if SEP_disp:
print("Symbols = %d, Errors %d, SEP = %1.2e" \
% (len(errors), len(idx), len(idx)/float(len(errors))))
return len(errors), len(idx), len(idx)/float(len(errors))
After Change
rx_data = np.rint((M-1)*(rx_data + (1+1j))/2.)
// Fix-up edge points real part
s1r = np.nonzero(np.ravel(rx_data.real > M - 1))[0]
s2r = np.nonzero(np.ravel(rx_data.real < 0))[0]
rx_data.real[s1r] = (M - 1)*np.ones(len(s1r))
rx_data.real[s2r] = np.zeros(len(s2r))
// Fix-up edge points imag part
s1i = np.nonzero(np.ravel(rx_data.imag > M - 1))[0]
s2i = np.nonzero(np.ravel(rx_data.imag < 0))[0]
rx_data.imag[s1i] = (M - 1)*np.ones(len(s1i))
rx_data.imag[s2i] = np.zeros(len(s2i))
rx_data = 2*rx_data - (M - 1)*(1 + 1j)
//Correlate the first Ncorr symbols at four possible phase rotations
R0,lags = xcorr(rx_data,tx_data,Ncorr)
R1,lags = xcorr(rx_data*(1j)**1,tx_data,Ncorr)
R2,lags = xcorr(rx_data*(1j)**2,tx_data,Ncorr)
R3,lags = xcorr(rx_data*(1j)**3,tx_data,Ncorr)
//Place the zero lag value in the center of the array
R0max = np.max(R0.real)
R1max = np.max(R1.real)
R2max = np.max(R2.real)
R3max = np.max(R3.real)
R = np.array([R0max,R1max,R2max,R3max])
Rmax = np.max(R)
kphase_max = np.where(R == Rmax)[0]
kmax = kphase_max[0]
//Find correlation lag value is zero at the center of the array
if kmax == 0:
lagmax = lags[np.where(R0.real == Rmax)[0]]
elif kmax == 1:
lagmax = lags[np.where(R1.real == Rmax)[0]]
elif kmax == 2:
lagmax = lags[np.where(R2.real == Rmax)[0]]
elif kmax == 3:
lagmax = lags[np.where(R3.real == Rmax)[0]]
taumax = lagmax[0]
if SEP_disp:
print("Phase ambiquity = (1j)**%d, taumax = %d" % (kmax, taumax))
//Count symbol errors over the entire input ndarrays
//Begin by making tx and rx length equal and apply
//phase rotation to rx_data
if taumax < 0:
tx_data = tx_data[-taumax:]
tx_data = tx_data[:min(len(tx_data),len(rx_data))]
rx_data = (1j)**kmax*rx_data[:len(tx_data)]
else:
rx_data = (1j)**kmax*rx_data[taumax:]
rx_data = rx_data[:min(len(tx_data),len(rx_data))]
tx_data = tx_data[:len(rx_data)]
//Convert QAM symbol difference to symbol errors
errors = np.int16(abs(rx_data-tx_data))
// Detect symbols errors
// Could decode bit errors from symbol index difference
idx = np.nonzero(np.ravel(errors != 0))[0]
if SEP_disp:
print("Symbols = %d, Errors %d, SEP = %1.2e" \
% (len(errors), len(idx), len(idx)/float(len(errors))))
return len(errors), len(idx), len(idx)/float(len(errors))
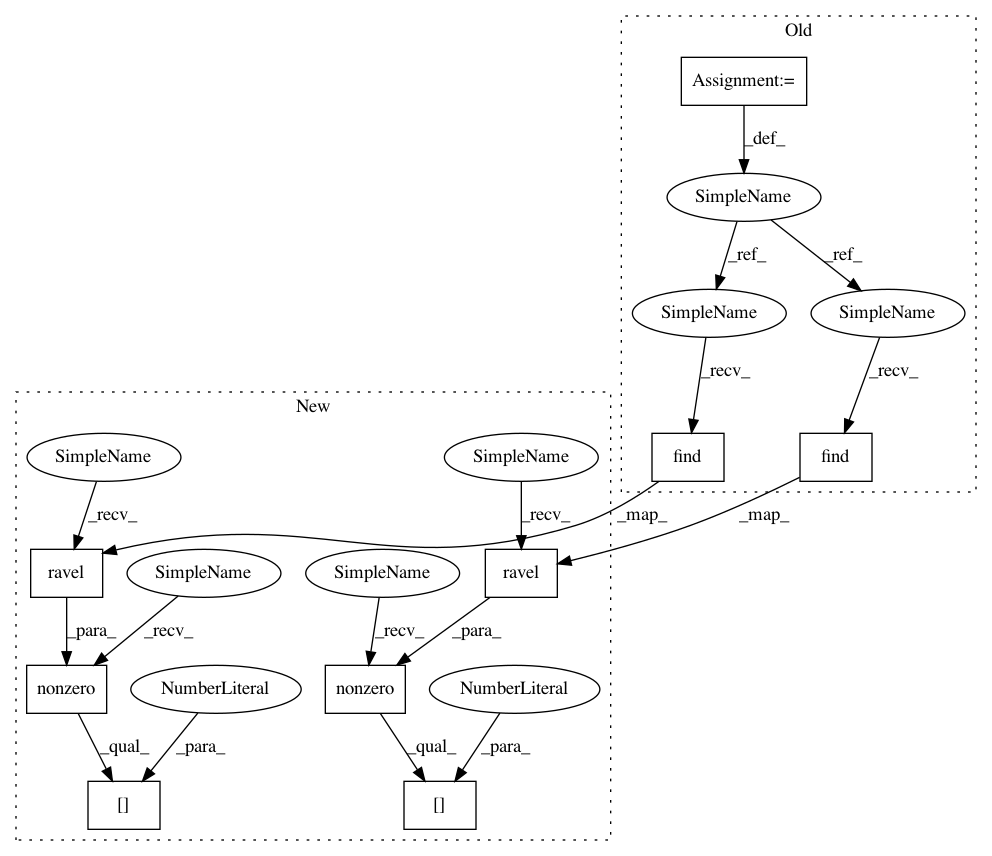
In pattern: SUPERPATTERN
Frequency: 6
Non-data size: 9
Instances
Project Name: mwickert/scikit-dsp-comm
Commit Name: 153f77827dd75fc74aff90838d0f6b3955c1b27d
Time: 2018-04-26
Author: Chiranth.Siddappa@cosmicaes.com
File Name: sk_dsp_comm/digitalcom.py
Class Name:
Method Name: QAM_SEP
Project Name: mwickert/scikit-dsp-comm
Commit Name: 153f77827dd75fc74aff90838d0f6b3955c1b27d
Time: 2018-04-26
Author: Chiranth.Siddappa@cosmicaes.com
File Name: sk_dsp_comm/digitalcom.py
Class Name:
Method Name: QAM_SEP
Project Name: mwickert/scikit-dsp-comm
Commit Name: 62e0bbfe37f9fda0556eab1d4a0333e14229f2ba
Time: 2018-11-28
Author: mwickert@uccs.edu
File Name: sk_dsp_comm/iir_design_helper.py
Class Name:
Method Name: sos_zplane
Project Name: mwickert/scikit-dsp-comm
Commit Name: 21616eefc617c2ec89e397776b20b0e891bddc0f
Time: 2018-04-28
Author: Chiranth.Siddappa@cosmicaes.com
File Name: sk_dsp_comm/sigsys.py
Class Name:
Method Name: splane
Project Name: mwickert/scikit-dsp-comm
Commit Name: 21616eefc617c2ec89e397776b20b0e891bddc0f
Time: 2018-04-28
Author: Chiranth.Siddappa@cosmicaes.com
File Name: sk_dsp_comm/sigsys.py
Class Name:
Method Name: zplane
Project Name: mwickert/scikit-dsp-comm
Commit Name: 21616eefc617c2ec89e397776b20b0e891bddc0f
Time: 2018-04-28
Author: Chiranth.Siddappa@cosmicaes.com
File Name: sk_dsp_comm/sigsys.py
Class Name:
Method Name: simpleQuant
Project Name: mwickert/scikit-dsp-comm
Commit Name: 5ccdb281e72eeb4e04a327a4dfdde0a07f5bfd9c
Time: 2018-11-03
Author: mwickert@uccs.edu
File Name: sk_dsp_comm/pyaudio_helper.py
Class Name: DSP_io_stream
Method Name: cb_active_plot