69638edca8afc4fef18c77c78171a808a7d359d6,trunk/SUAVE/Methods/Performance/estimate_take_off_field_length.py,,estimate_take_off_field_length,#Any#Any#Any#Any#,31
Before Change
// ==============================================
// Getting engine thrust
// ==============================================
state = Data()
state.conditions = Aerodynamics()
state.numerics = Numerics()
conditions = state.conditions
conditions.freestream.dynamic_pressure = np.array(np.atleast_1d(0.5 * rho * speed_for_thrust**2))
conditions.freestream.gravity = np.array([np.atleast_1d(sea_level_gravity)])
conditions.freestream.velocity = np.array(np.atleast_1d(speed_for_thrust))
conditions.freestream.mach_number = np.array(np.atleast_1d(speed_for_thrust/ a))
conditions.freestream.speed_of_sound = np.array(a)
conditions.freestream.temperature = np.array(np.atleast_1d(T))
conditions.freestream.pressure = np.array(np.atleast_1d(p))
conditions.propulsion.throttle = np.array(np.atleast_1d(1.))
results = vehicle.propulsors.evaluate_thrust(state) // total thrust
thrust = results.thrust_force_vector
// ==============================================
// Calculate takeoff distance
// ==============================================
// Defining takeoff distance equations coefficients
try:
takeoff_constants = vehicle.takeoff_constants // user defined
except: // default values
takeoff_constants = np.zeros(3)
if engine_number == 2:
takeoff_constants[0] = 857.4
takeoff_constants[1] = 2.476
takeoff_constants[2] = 0.00014
elif engine_number == 3:
takeoff_constants[0] = 667.9
takeoff_constants[1] = 2.343
takeoff_constants[2] = 0.000093
elif engine_number == 4:
takeoff_constants[0] = 486.7
takeoff_constants[1] = 2.282
takeoff_constants[2] = 0.0000705
elif engine_number > 4:
takeoff_constants[0] = 486.7
takeoff_constants[1] = 2.282
takeoff_constants[2] = 0.0000705
print("The vehicle has more than 4 engines. Using 4 engine correlation. Result may not be correct.")
else:
takeoff_constants[0] = 857.4
takeoff_constants[1] = 2.476
takeoff_constants[2] = 0.00014
print("Incorrect number of engines: {0:.1f}. Using twin engine correlation.".format(engine_number))
// Define takeoff index (V2^2 / (T/W)
takeoff_index = V2_speed**2. / (thrust[0][0] / weight)
// Calculating takeoff field length
takeoff_field_length = 0.
for idx,constant in enumerate(takeoff_constants):
takeoff_field_length += constant * takeoff_index**idx
takeoff_field_length = takeoff_field_length * Units.ft
// calculating second segment climb gradient, if required by user input
if compute_2nd_seg_climb:
// Getting engine thrust at V2 (update only speed related conditions)
state.conditions.freestream.dynamic_pressure = np.array(np.atleast_1d(0.5 * rho * V2_speed**2))
state.conditions.freestream.velocity = np.array(np.atleast_1d(V2_speed))
state.conditions.freestream.mach_number = np.array(np.atleast_1d(V2_speed/ a))
results = vehicle.propulsors["turbofan"].engine_out(state)
thrust = results.thrust_force_vector[0][0]
// Compute windmilling drag
windmilling_drag_coefficient = windmilling_drag(vehicle,state)
// Compute asymmetry drag
asymmetry_drag_coefficient = asymmetry_drag(state, vehicle, windmilling_drag_coefficient)
// Compute l over d ratio for takeoff condition, NO engine failure
l_over_d = estimate_2ndseg_lift_drag_ratio(vehicle)
// Compute L over D ratio for takeoff condition, WITH engine failure
clv2 = maximum_lift_coefficient / (V2_VS_ratio) **2
cdv2_all_engine = clv2 / l_over_d
cdv2 = cdv2_all_engine + asymmetry_drag_coefficient + windmilling_drag_coefficient
l_over_d_v2 = clv2 / cdv2
// Compute 2nd segment climb gradient
second_seg_climb_gradient = thrust / (weight*sea_level_gravity) - 1. / l_over_d_v2
return takeoff_field_length, second_seg_climb_gradient
else:
// return only takeoff_field_length
return takeoff_field_length
After Change
// Compute 2nd segment climb gradient
second_seg_climb_gradient = thrust / (weight*sea_level_gravity) - 1. / l_over_d_v2
return takeoff_field_length[0][0], second_seg_climb_gradient[0][0]
else:
// return only takeoff_field_length
return takeoff_field_length[0][0]
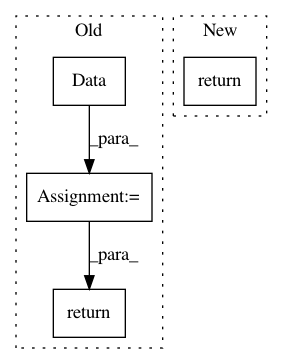
In pattern: SUPERPATTERN
Frequency: 3
Non-data size: 4
Instances
Project Name: suavecode/SUAVE
Commit Name: 69638edca8afc4fef18c77c78171a808a7d359d6
Time: 2020-04-14
Author: ebotero@stanford.edu
File Name: trunk/SUAVE/Methods/Performance/estimate_take_off_field_length.py
Class Name:
Method Name: estimate_take_off_field_length
Project Name: rusty1s/pytorch_geometric
Commit Name: 5718673905b27722d458f70de66d8556f369e341
Time: 2018-04-19
Author: matthias.fey@tu-dortmund.de
File Name: torch_geometric/data/in_memory_dataset.py
Class Name: InMemoryDataset
Method Name: get_data
Project Name: suavecode/SUAVE
Commit Name: 5678e66aaa00587902c71449bf87270e9d07384f
Time: 2019-03-16
Author: mclarke2@stanford.edu
File Name: trunk/SUAVE/Methods/Aerodynamics/XFOIL/compute_airfoil_polars.py
Class Name:
Method Name: read_airfoil_polars