a9b528c9bdeeb76d390827d4efdad4945f5c424c,examples/cifar_adversarial_training.py,,,#,10
Before Change
classifier.fit(x_train, y_train, nb_epochs=10, batch_size=128)
// Craft adversarial samples with DeepFool
print("\nCreate DeepFool attack")
adv_crafter = DeepFool(classifier)
print("\nCraft attack on training examples")
x_train_adv = adv_crafter.generate(x_train)
print("\nCraft attack test examples")
After Change
from art.utils import load_dataset
// Configure a logger to capture ART outputs; these are printed in console and the level of detail is set to INFO
logger = logging.getLogger()
logger.setLevel(logging.INFO)
handler = logging.StreamHandler()
formatter = logging.Formatter("[%(levelname)s] %(message)s")
handler.setFormatter(formatter)
logger.addHandler(handler)
// Read CIFAR10 dataset
(x_train, y_train), (x_test, y_test), min_, max_ = load_dataset(str("cifar10"))
x_train, y_train = x_train[:5000], y_train[:5000]
x_test, y_test = x_test[:500], y_test[:500]
im_shape = x_train[0].shape
// Create Keras convolutional neural network - basic architecture from Keras examples
// Source here: https://github.com/keras-team/keras/blob/master/examples/cifar10_cnn.py
k.set_learning_phase(1)
model = Sequential()
model.add(Conv2D(32, (3, 3), padding="same", input_shape=x_train.shape[1:]))
model.add(Activation("relu"))
model.add(Conv2D(32, (3, 3)))
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Conv2D(64, (3, 3), padding="same"))
model.add(Activation("relu"))
model.add(Conv2D(64, (3, 3)))
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(512))
model.add(Activation("relu"))
model.add(Dropout(0.5))
model.add(Dense(10))
model.add(Activation("softmax"))
model.compile(loss="categorical_crossentropy", optimizer="adam", metrics=["accuracy"])
// Create classifier wrapper
classifier = KerasClassifier((min_, max_), model=model)
classifier.fit(x_train, y_train, nb_epochs=10, batch_size=128)
// Craft adversarial samples with DeepFool
logger.info("Create DeepFool attack")
adv_crafter = DeepFool(classifier)
logger.info("Craft attack on training examples")
x_train_adv = adv_crafter.generate(x_train)
logger.info("Craft attack test examples")
x_test_adv = adv_crafter.generate(x_test)
// Evaluate the classifier on the adversarial samples
preds = np.argmax(classifier.predict(x_test_adv), axis=1)
acc = np.sum(preds == np.argmax(y_test, axis=1)) / y_test.shape[0]
logger.info("Classifier before adversarial training")
logger.info("Accuracy on adversarial samples: %.2f%%", (acc * 100))
// Data augmentation: expand the training set with the adversarial samples
x_train = np.append(x_train, x_train_adv, axis=0)
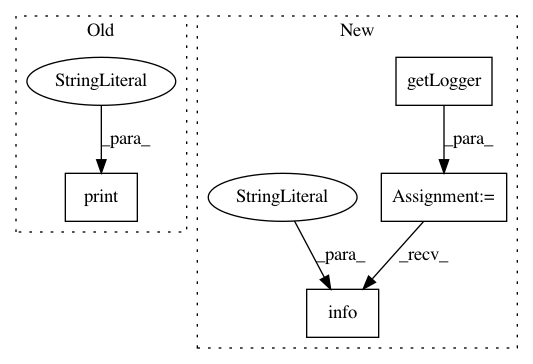
In pattern: SUPERPATTERN
Frequency: 5
Non-data size: 4
Instances
Project Name: IBM/adversarial-robustness-toolbox
Commit Name: a9b528c9bdeeb76d390827d4efdad4945f5c424c
Time: 2019-02-12
Author: Maria-Irina.Nicolae@ibm.com
File Name: examples/cifar_adversarial_training.py
Class Name:
Method Name:
Project Name: SeldonIO/seldon-core
Commit Name: d3874384937c4b5d50ac1e0b6f95c5bfe9be3ba1
Time: 2018-12-11
Author: jk@seldon.io
File Name: python/seldon_core/persistence.py
Class Name:
Method Name: restore
Project Name: IBM/adversarial-robustness-toolbox
Commit Name: 260185d588c91aa37991a9e3c886bf6ae2e57193
Time: 2019-02-13
Author: Maria-Irina.Nicolae@ibm.com
File Name: examples/cifar_adversarial_training.py
Class Name:
Method Name:
Project Name: sentinel-hub/eo-learn
Commit Name: 97617b16f041a0cdf13d2845c8f7c79569d72dc3
Time: 2018-08-02
Author: matej.aleksandrov@sinergise.com
File Name: core/eolearn/core/eoexecution.py
Class Name:
Method Name:
Project Name: tensorflow/tensorboard
Commit Name: fd1cdbfebb3ddedc6d96884995e8b4fd2bb292e3
Time: 2017-07-06
Author: jart@google.com
File Name: tensorboard/main.py
Class Name:
Method Name: run_simple_server