f93beff338925cc1bf1b3ff1b32a2c440a4c9427,tests/_tests_scripts/z_mvp_mnist_gan.py,CustomRunner,_handle_batch,#CustomRunner#Any#,18
Before Change
// fake
generated_labels_ = torch.zeros(bs, 1).to(self.device)
generated_pred_ = self.model["discriminator"](
generated_images.detach()
)
fake_loss = F.binary_cross_entropy(generated_pred_, generated_labels_)
// loss
loss_discriminator = (real_loss + fake_loss) / 2.0
self.state.batch_metrics["loss_discriminator"] = loss_discriminator
def main():
After Change
class CustomRunner(dl.Runner):
def _handle_batch(self, batch):
real_images, _ = batch
batch_metrics = {}
// Sample random points in the latent space
batch_size = real_images.shape[0]
random_latent_vectors = torch.randn(batch_size, latent_dim).to(
self.device
)
// Decode them to fake images
generated_images = self.model["generator"](
random_latent_vectors
).detach()
// Combine them with real images
combined_images = torch.cat([generated_images, real_images])
// Assemble labels discriminating real from fake images
labels = torch.cat(
[torch.ones((batch_size, 1)), torch.zeros((batch_size, 1))]
).to(self.device)
// Add random noise to the labels - important trick!
labels += 0.05 * torch.rand(labels.shape).to(self.device)
// Train the discriminator
predictions = self.model["discriminator"](combined_images)
batch_metrics[
"loss_discriminator"
] = F.binary_cross_entropy_with_logits(predictions, labels)
// Sample random points in the latent space
random_latent_vectors = torch.randn(batch_size, latent_dim).to(
self.device
)
// Assemble labels that say "all real images"
misleading_labels = torch.zeros((batch_size, 1)).to(self.device)
// Train the generator
generated_images = self.model["generator"](random_latent_vectors)
predictions = self.model["discriminator"](generated_images)
batch_metrics["loss_generator"] = F.binary_cross_entropy_with_logits(
predictions, misleading_labels
)
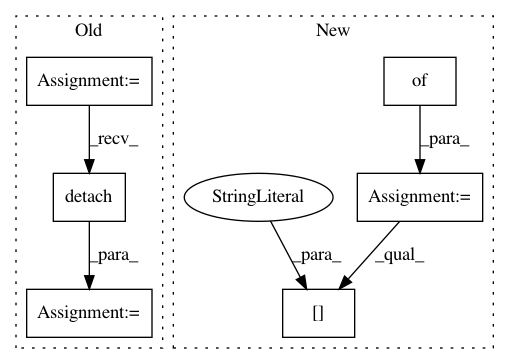
In pattern: SUPERPATTERN
Frequency: 3
Non-data size: 6
Instances
Project Name: Scitator/catalyst
Commit Name: f93beff338925cc1bf1b3ff1b32a2c440a4c9427
Time: 2020-04-21
Author: scitator@gmail.com
File Name: tests/_tests_scripts/z_mvp_mnist_gan.py
Class Name: CustomRunner
Method Name: _handle_batch
Project Name: OpenNMT/OpenNMT-py
Commit Name: e7d0f85e965deddd217aaa64728637173f1491d1
Time: 2017-06-14
Author: srush@seas.harvard.edu
File Name: onmt/Models.py
Class Name: Decoder
Method Name: forward