3218a3987ef9f83d1ad5efd6f96d6f6c741c3cfa,python/eight_mile/pytorch/layers.py,,viterbi,#Any#Any#Any#Any#Any#Any#,776
Before Change
seq_len, batch_size, tag_size = unary.size()
min_length = torch.min(lengths)
batch_range = torch.arange(batch_size, dtype=torch.int64)
backpointers = []
// Alphas: [B, 1, N]
alphas = torch.Tensor(batch_size, 1, tag_size).fill_(-1e4).to(unary.device)
alphas[:, 0, start_idx] = 0
alphas = F.log_softmax(alphas, dim=-1) if norm else alphas
for i, unary_t in enumerate(unary):
next_tag_var = alphas + trans
viterbi, best_tag_ids = torch.max(next_tag_var, 2)
backpointers.append(best_tag_ids.data)
new_alphas = viterbi + unary_t
new_alphas.unsqueeze_(1)
if i >= min_length:
mask = (i < lengths).view(-1, 1, 1)
alphas = alphas.masked_fill(mask, 0) + new_alphas.masked_fill(mask == 0, 0)
else:
alphas = new_alphas
// Add end tag
terminal_var = alphas.squeeze(1) + trans[:, end_idx]
_, best_tag_id = torch.max(terminal_var, 1)
path_score = terminal_var[batch_range, best_tag_id] // Select best_tag from each batch
best_path = [best_tag_id]
// Flip lengths
rev_len = seq_len - lengths - 1
for i, backpointer_t in enumerate(reversed(backpointers)):
// Get new best tag candidate
new_best_tag_id = backpointer_t[batch_range, best_tag_id]
// We are going backwards now, if you passed your flipped length then you aren"t in your real results yet
mask = (i > rev_len)
best_tag_id = best_tag_id.masked_fill(mask, 0) + new_best_tag_id.masked_fill(mask == 0, 0)
best_path.append(best_tag_id)
_ = best_path.pop()
best_path.reverse()
best_path = torch.stack(best_path)
// Return list of paths
paths = []
best_path = best_path.transpose(0, 1)
for path, length in zip(best_path, lengths):
paths.append(path[:length])
return paths, path_score.squeeze(0)
class TaggerGreedyDecoder(nn.Module):
After Change
best_path = torch.stack(best_path)
// Mask out the extra tags (This might be pointless given that anything that
// will use this as a dense tensor downstream will mask it itself?)
seq_mask = sequence_mask(lengths).to(best_path.device).transpose(0, 1)
best_path = best_path.masked_fill(seq_mask == 0, 0)
return best_path, path_score
class TaggerGreedyDecoder(nn.Module):
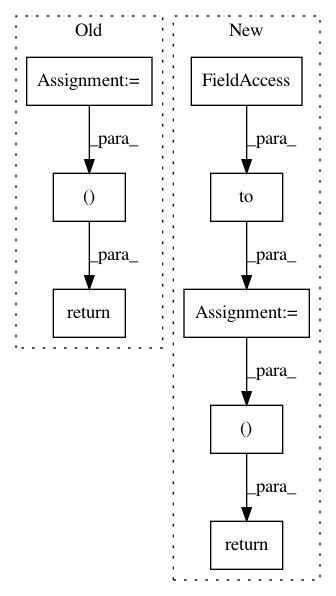
In pattern: SUPERPATTERN
Frequency: 3
Non-data size: 8
Instances
Project Name: dpressel/mead-baseline
Commit Name: 3218a3987ef9f83d1ad5efd6f96d6f6c741c3cfa
Time: 2019-09-27
Author: dpressel@gmail.com
File Name: python/eight_mile/pytorch/layers.py
Class Name:
Method Name: viterbi
Project Name: HyperGAN/HyperGAN
Commit Name: ee19c97fefd74ce588428a96e157bb826c0a450c
Time: 2020-12-20
Author: martyn@255bits.com
File Name: hypergan/samplers/aligned_sampler.py
Class Name: AlignedSampler
Method Name: _sample
Project Name: dpressel/mead-baseline
Commit Name: 9b72ec0d4963412e9790b06d22f051a9723af33c
Time: 2019-02-24
Author: blester125@users.noreply.github.com
File Name: python/baseline/pytorch/crf.py
Class Name:
Method Name: viterbi